Learn Python with our free tutorial, suitable for beginners. It contains carefully crafted, logically ordered Python articles full of information, advice, and Python practice! Hence, it helps both complete beginners and those with prior programming experience get up to speed with Python.
If you want to earn a certificate, track your progress, and have access to premium support, please consider our Python Fundamentals course.
Table of Contents
How to learn Python?
If you’re in a hurry to learn Python, I’ll give you some shortcuts to get you started quickly. You have two options:
- Our premium Python course, Python Fundamentals, is the fastest and easiest way to learn Python. It is designed to teach Python quickly but properly, without distractions, using lots of quizzes, exercises, and a certificate of completion that you can add to your resume.
- Use the free Python tutorial that we kick off right on this page. Simply keep reading. You can always decide to join the course at a later moment.
Why learn Python?
Since you’re here, you probably know why, but let’s quickly review Python’s advantages!
Python is one of the world’s most used and most popular programming languages. It’s powerful, versatile, and easy to learn. Python is widely used in various applications, some notable ones:
- Web development
- Data Science
- Data analysis
- Machine learning
- Artificial Intelligence (AI)
- Scripting and tooling
Many people say that Python comes with batteries included. It’s a fun way to state that it includes a comprehensive base library. In addition, because so many people use Python, hundreds of thousands of high-quality libraries and frameworks exist to get things done quickly and without hassle. You can do a lot with a little bit of Python code!
Learning Python is a no-brainer, and I promise you will be up and running quickly with this Python tutorial. Regardless of your future in IT, it will be a helpful tool to have in your toolbox!
Why this Python tutorial?
And here’s why you should read this Python tutorial instead of all the others:
- This free Python tutorial is easy to read and is in plain English.
- It’s written by an experienced writer and tutor who puts great care into the learning material and the order in which it is presented.
- This course contains interactive example code you can edit and run. It’s great fun and helps you to learn concepts much faster.
- This course is practical. While focussing on getting stuff done in the real world, I also explain how and why things work instead of teaching you tricks.
- It provides carefully vetted links on most pages to deepen your knowledge.
- Did I mention it’s completely free, with no strings attached? We offer premium Python courses for those looking for a premium experience, extra practice, and a certificate of completion.
About the instructor
So, what makes me eligible to teach you Python? Let me introduce myself!
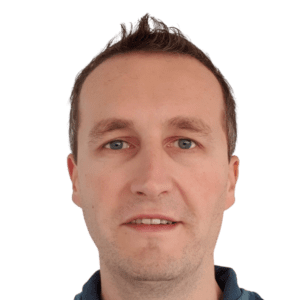
I’m Erik, and I’ve worked as a software engineer for over 25 years. I hold an MSc in computer science and used many programming languages in my career, but Python is my absolute favorite! Although I love programming and building complex systems, I also love writing and teaching. Hence, I combined these two by writing tutorials and courses on this site.
Eventually, I got fed up with the limited copy-and-paste code examples and wanted example code that is editable and runnable in-page. It resulted in a side project (crumb.sh) that offers a generic way to do this. The courses have many valuable code crumbs sprinkled throughout them to improve the learning experience!
Stay up-to-date
If you’re on Twitter, you can follow me (@erikyan) to get updates on new content.
If you prefer e-mail, try my weekly Python newsletter. You can subscribe here or at the bottom of any page. I use it to:
- Inform you about current Python trends
- New and existing articles you might like
- I’ll include occasional course discount codes, too.
Python history
Let’s start by defining Python more precisely. Python is a computer programming language. Or, in other words, a vocabulary and set of grammatical rules for instructing a computer to perform tasks. Its original creator, Guido van Rossum, named it after the BBC television show ‘Monty Python’s Flying Circus.’ Hence, you’ll find that Python books, code examples, and documentation sometimes contain references to this television show.
In 1987, Guido worked on a large distributed operating system at the CWI, a national research institute for mathematics and computer science in the Netherlands. Within that project, he had some freedom to work on side projects. With the knowledge and experience he had built up in the years before, working on a computer language called ABC, he started writing the Python programming language.
Python is easy to learn, and it’s designed around a set of clearly defined principles (the Zen of Python) that encourage Python core developers to make a language that is unambiguous and easy to use.
Extensible
In a 2003 interview with Bill Venners, Guido mentioned what was probably the biggest innovation in the new language:
I think my most innovative contribution to Python’s success was making it easy to extend. That also came out of my frustration with ABC. ABC was a very monolithic design. There was a language design team, and they were God. They designed every language detail and there was no way to add to it. You could write your own programs, but you couldn’t easily add low-level stuff.
Guido van Rossum
He decided you should be able to extend the language in two ways: by writing pure Python modules or by writing a module entirely in C. The former is obviously easier, but the latter allows for fast, high-performance modules like NumPy. It was a success because his CWI colleagues, the users, and Guido himself immediately started writing their own extension modules. The extension modules let you do all sorts of things. Just a small selection of modules that exist today:
- graphics libraries,
- data processing and data science libraries like NumPy and Pandas,
- AI and machine learning libraries,
- libraries to work with all sorts of file formats (like JSON, YAML),
- all kinds of libraries to use and connect external services,
- build websites and website backends (e.g., Django, FastAPI)
- … and so on
Since its inception, Guido has been actively involved in Python’s development. After a short retirement, he returned to work. Microsoft employs him, and his main focus is improving Python’s speed.
A timeline
The following figure shows a global timeline of Python’s historical and most defining releases:
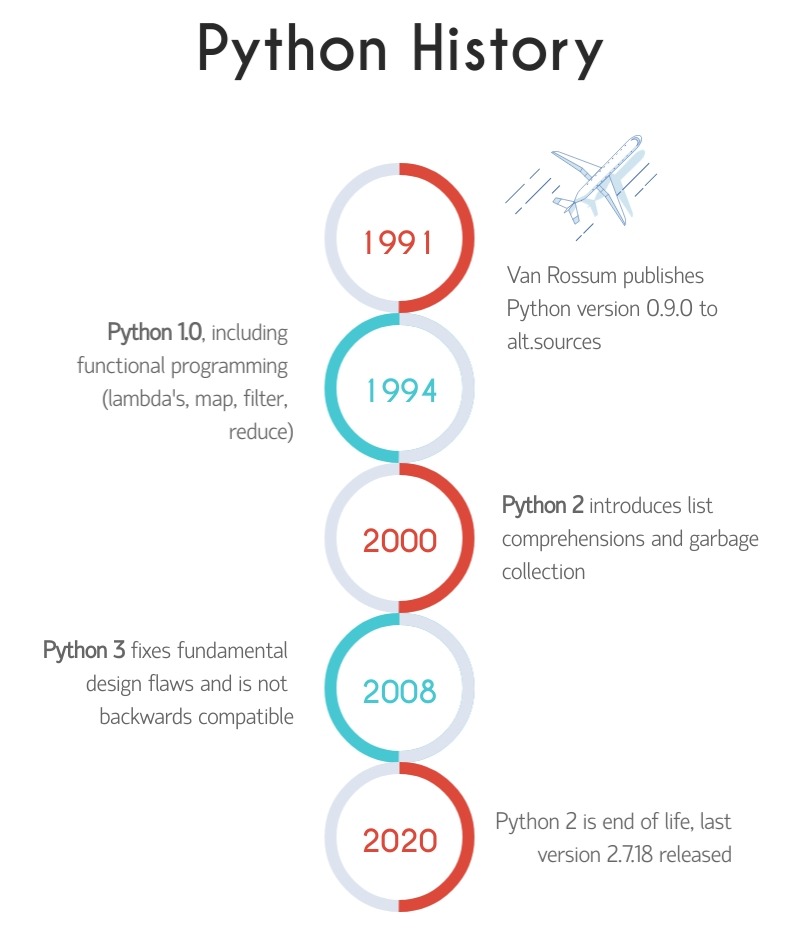
Python 2 vs. Python 3
As you can see from the Python history timeline, Python 2 and 3 have been developed and maintained side by side for an extended period. The primary reason is that Python 3 code is not entirely backward compatible with Python 2 code. This incompatibility caused a prolonged adoption rate. Many people were happy with version 2 and had no reason to upgrade.
On top of that, Python 3 was initially slower than Python 2. As Python 3 kept improving and receiving new features, it eventually took off. With the latest efforts led by Guido, Python 3 is now faster than ever. In addition, Python 3 adds many useful features to the language, making it easier and more fun. Unless you need to maintain a legacy code base, avoid Python 2.
Run Your First Python Program
Let’s dive in directly! We’ll run the Python code from your browser to get started quickly. Later, you’ll learn how to install Python on your computer.
You can use the Python Playground to test and experiment with the examples in this Python tutorial. It allows you to enter Python code and run it by pressing the big green play button. The code runs in our backend on a real computer. Try it!
Hello World
There’s a tradition in which programming tutorials, books, and courses start with a so-called Hello World program. A Hello World program simply prints the words “Hello world” to the screen. The Python playground above does precisely this, using the print()
function.
The print()
function takes anything you put between the parentheses and prints it to the screen. But we must feed it the correct type of data for it to work. For example, we need to put text in Python between quotes. In the world of computer programming, we call this a string.
Quotes around strings are essential because they precisely mark the start and end of a string. This way, a string is easy to recognize for Python. Here are a few more examples of valid strings:
'Hello world'
'My name is Eric'
'This one is a bit longer.'
Code language: Python (python)
You can print these strings or a string of your choosing in the Python playground above, and I encourage you to do so! Like most things in life, programming requires practice to master it.
I did everything I could to make browsing the free Python tutorial as easy as can be! Primarily, you can use the top menu. In addition, navigational links at the top and end of each page guide you to the next topic or return to the previous one. I also link to related pages to deepen your knowledge.
I carefully ordered the course topics so that you can start from the beginning and work your way up. However, feel free to browse around!
How can you help me?
You—yes, that’s you—can help me improve this course in several ways.
1. Get in touch if you…
- find any mistakes,
- think something can be improved,
- or something is unclear to you.
2. Donate or buy the course
I’ve been working on this site for about three years, spending most of my spare time here with all my heart and soul. It must have been 1000s of hours by now. I hope it shows, and I genuinely hope you have a lot of fun learning Python here.
Buying my Python course for beginners is the best way to support my work. If that’s not an option and you still want to show your appreciation, you can buy me a coffee. All the support I have gotten so far encourages me to keep writing and updating the content!
3. Follow me
You can follow me on Bluesky for updates on new content, links, quizzes, code snippets, etc. This site also has a dedicated mailing list; subscribe to my newsletter. It’s low-volume and mainly contains new articles I wrote, interesting links to read, and occasional discounts for my premium courses!
Let’s go and learn Python!
Ready to learn Python? To follow this tutorial, use the navigational buttons at the bottom and top of each page, or browse around using the site menu on the right (or top if you have a small screen). If you have any questions, don’t hesitate to contact me through the contact form.