Jupyter Notebook
Before starting with NumPy, I’d like to introduce you to Jupyter Notebook, part of the Jupyter Lab interactive IDE ideal for scientific and interactive computing. We’ll explore its advantages over a regular IDE, show you how to install Jupyter Notebook and Jupyter Lab, and demonstrate its abilities.
Jupyter Lab vs Jupyter Notebook
JupyterLab is a web-based, interactive development environment. It’s most well known for offering a so-called notebook called Jupyter Notebook, but you can also use it to create and edit other files, like code, text files, and markdown files. In addition, it allows you to open a Python terminal, as most IDEs do, to experiment and tinker.
JupyterLab is flexible: you can configure and arrange the user interface to support a wide range of data science, scientific computing, and machine learning workflows. It’s also extensible: everyone can write plugins that add new components and integrate with existing ones.
JupyterLab supports over 40 programming languages like Java, Scala, Julia, and R. However, I’ll focus solely on Python in this article.
On the other hand, Jupyter Notebook is a REPL-like environment that fuses code, data, and documentation. So in short, JupyterLab is the browser-based IDE, while Jupyter Notebook is the Notebook component. You can install Jupyter Notebook separately, and I’ll show you how in this article.
Advantages of using Jupyter Lab and Notebook
Python application developers often prefer and work with a regular Python IDE like VSCode, which facilitates debugging, unit testing, deployment, and version management. In contrast, (data) scientists, data analysts, and machine learning/AI people have a different focus and often prefer a Notebook-style IDE. Let’s look at some of the advantages these notebooks have to offer.
Combine and document code and results
The biggest advantage of using notebooks is that it allows you to combine three things into one environment:
- documentation,
- code,
- results
All the while offering an interactive environment where you or your fellows can tinker and experiment. The following image perfectly demonstrates this:
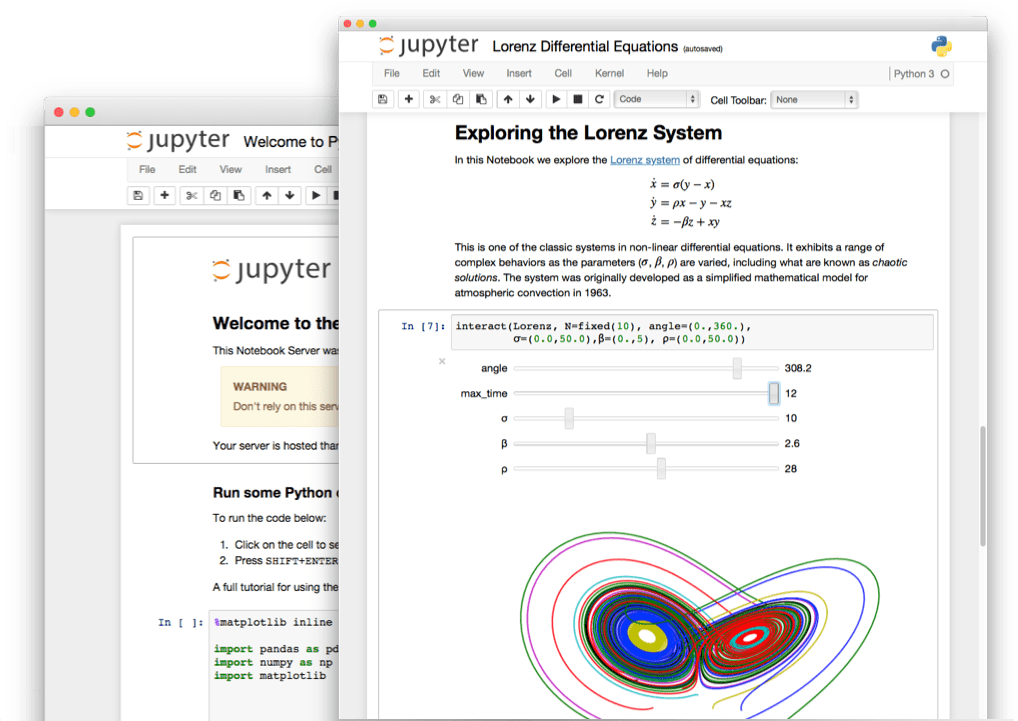
Interactive exploration
A Notebook allows you to explore your data interactively. While doing so, the Notebook is a log of what you did and how you did it. It’s ideal to use in combination with NumPy and Pandas. So a Notebook is much more geared toward those working with and analyzing data than regular IDEs. However, some IDEs, like VSCode, will offer a built-in Jupyter Notebook (see further down this article).
It’s what the others use
Sometimes it helps to go with the flow. In this case, you’ll notice that many others in data science use Jupyter Notebooks. This has a couple of advantages on its own:
- It’s easy to exchange and share your work.
- Many plugins are created by fellow data scientists, specifically targeted at people like you.
How to install Jupyter Notebook and Lab
Like all Python software, installing Jupyter Lab is a breeze. I’ll demonstrate how to install with both Pip and Conda. One thing to note beforehand: I recommend installing this system-wide. I normally stress the importance of using a virtual environment. However, I feel that tools like this you want to install once.
However, if you get issues with Jupyter not being in your PATH, installing it in a virtual environment may be easier.
Install Jupyter Lab and/or Notebook with Pip
As noted above, you want to install Jupyter Lab system-wide or at least in your local bin folder. Installing the package globally with Pip requires the -g
option. On many Linux systems, however, Pip will install the package in a .local/bin
folder if you don’t specify -g and if you’re not using a virtual environment. You can force this behavior by using the --user
option. The advantage is that you don’t need root access to the system you’re working on.
So, the most recommended way to install Jupyter Lab is to use the pip install command:
$ pip install --user jupyterlab
If you only want Jupyter Notebook, use this instead:
$ pip install --user notebook
Install JupyterLab with Conda
JupyterLab can be installed with mamba
and conda
as well:
$ mamba install -c conda-forge jupyterlab or... $ conda install -c conda-forge jupyterlab
Like with Pip, if you only want o install Jupyter Notebook, use this:
$ mamba install -c conda-forge notebook or... $ conda install -c conda-forge notebook
Start and explore JupyterLab
How to open Jupyter Lab from the command-line
If you followed the installation instructions, you should be able to start Jupyter Lab with:
$ jupyter-lab (a bunch of log message will follow)
How to open Jupyter Notebook from the command-line
If you only want to start a Notebook, use this:
$ jupyter-notebook (a bunch of log message will follow)
Adding to your PATH
If you get an error like “command not found,” don’t panic. Unfortunately, some systems don’t have the .local/bin
directory in the $PATH
variable.
Windows
On Windows, you need to look carefully at the output of your installer. In my case, pip warned me that the binaries were not on the PATH. It also hinted to me to add this atrocious path to the PATH:
C:\Users\erik\AppData\Local\Packages\PythonSoftwareFoundation.Python.3.10_qbz5n2kfra8p0\LocalCache\local-packages\Python310\Scripts
It will almost certainly be a different path for you. And if you change Python versions, this will also change, I’m afraid. If you don’t know how to add something to your Windows PATH, please use Google. There are several good guides out there. Alternatively, I recommend installing this in a virtual environment.
Linux and Mac
On Linux and OS X, a quick fix is to add it temporarily like this:
PATH="$HOME/.local/bin:$PATH"
However, this will only work as long as this terminal window is open. So, to make this permanent, I recommend adding the following snippet to the end of your ~/.profile
file:
# set PATH so it includes user's private bin if it exists if [ -d "$HOME/.local/bin" ] ; then PATH="$HOME/.local/bin:$PATH" fi
All it does is add the .local/bin folder to the PATH, but only if it exists.
The .profile
file is loaded once when you log in to your system. You probably need to log out and log in to apply the changes. In case you use zsh instead of Bash (zsh is the default on MacOS these days), you may want to try adding the snippet about to a file called .zprofile
instead.
Your first steps in a Notebook
If you start Jupyter Lab or Notebook, it will automatically open your browser. If it didn’t, you can go to http://localhost:8888/ manually to open it. Once there, create a new Notebook, e.g., by clicking File -> New -> Notebook.
I created a new Notebook named ‘First steps’ in the following screenshot. There are a couple of noteworthy things to see:
- Your input is placed in a ‘cell,’ and each cell is numbered
- You can access the previous cells by using the
In
andOut
arrays. E.g.,Out[3]
in the example below gives us the output of the expression in theIn[3]
cell - You can also change the cell type to Markdown; for more on this, see below
- If you want to apply or execute your cell, press Shift + Enter.
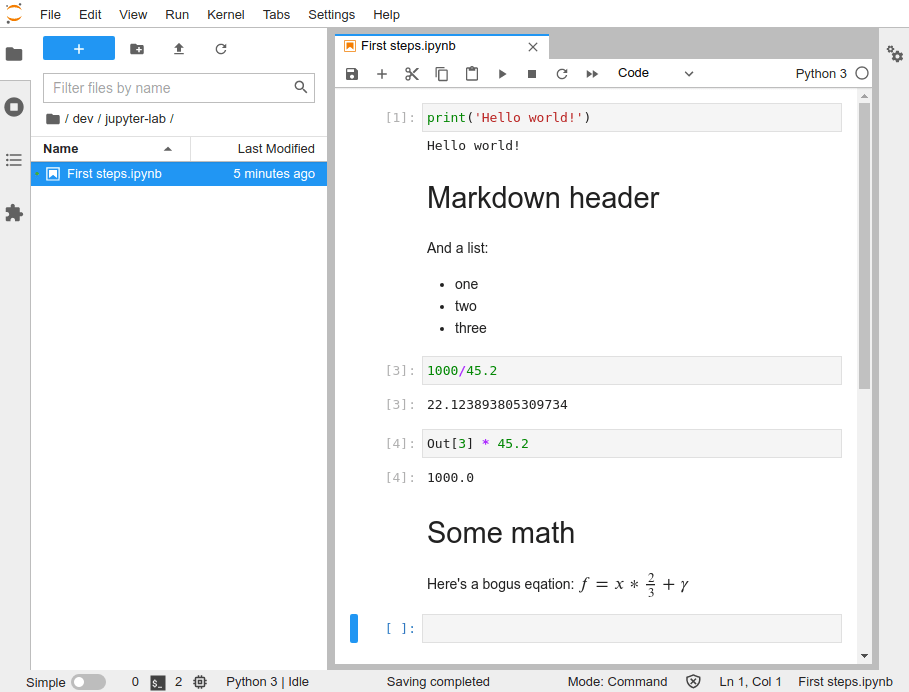
Cell types
A dropdown at the top of your notebook allows you to change the cell type. All you need to care about, for now, are the types ‘Python’ and ‘Markdown.’
Python
A Python cell contains Python code. This can be any valid code, so all these are allowed:
- One simple line of code
- A Python import statement
- A function definition
- A complete class definition
- Etcetera!
Markdown
Markdown is a lightweight markup language for creating formatted text. You can use it to create headers, lists, code snippets, and most other things you can think of. For example, you might be familiar with it if you have ever created a README.md file on GitHub.
Markdown is great for adding documentation to your notebook. For most, using a Markdown cheat sheet is enough to get started quickly.
Formulas
You can enter nice-looking formulas using Markdown as well. Internally, Jupyter uses MathJax to format and display formulas. A formula starts and ends with either a single or a double dollar sign:
$ .... $
: in-line; the formula is in line with the rest of the current sentence$$ .. $$
: display mode; the formula stands out from the rest of the text
Inside, you need to enter a TeX-based equation. If you want to see all the options and play around with such equations, I recommend this website that offers a Tex equation editor.
We use the same MathJax library to display formulas on this site. You can right-click on a formula to get more options and copy the Tex equivalent for use in Markdown. You can try this on the formula below:
\(y = x * 5\)JupyterLab plugins
If you click on the puzzle icon at the left of the screen, you’ll get a list of plugins that can be installed directly. There are lots of plugins, and many of them will add extra visualization capabilities or integration with products like Tableau.
Jupyter Notebook in VSCode
If you’re a VSCode fan, like me, you need to check out the VSCode extension that allows you to create and work with notebooks directly inside a VSCode tab. If you open up the command pallet, e.g., with ctrl + shift + p, start typing “Jupyter” to create, open, and export Notebooks.
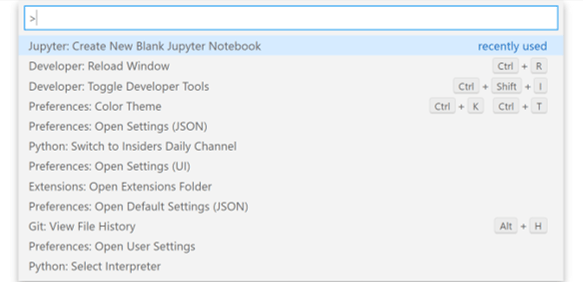